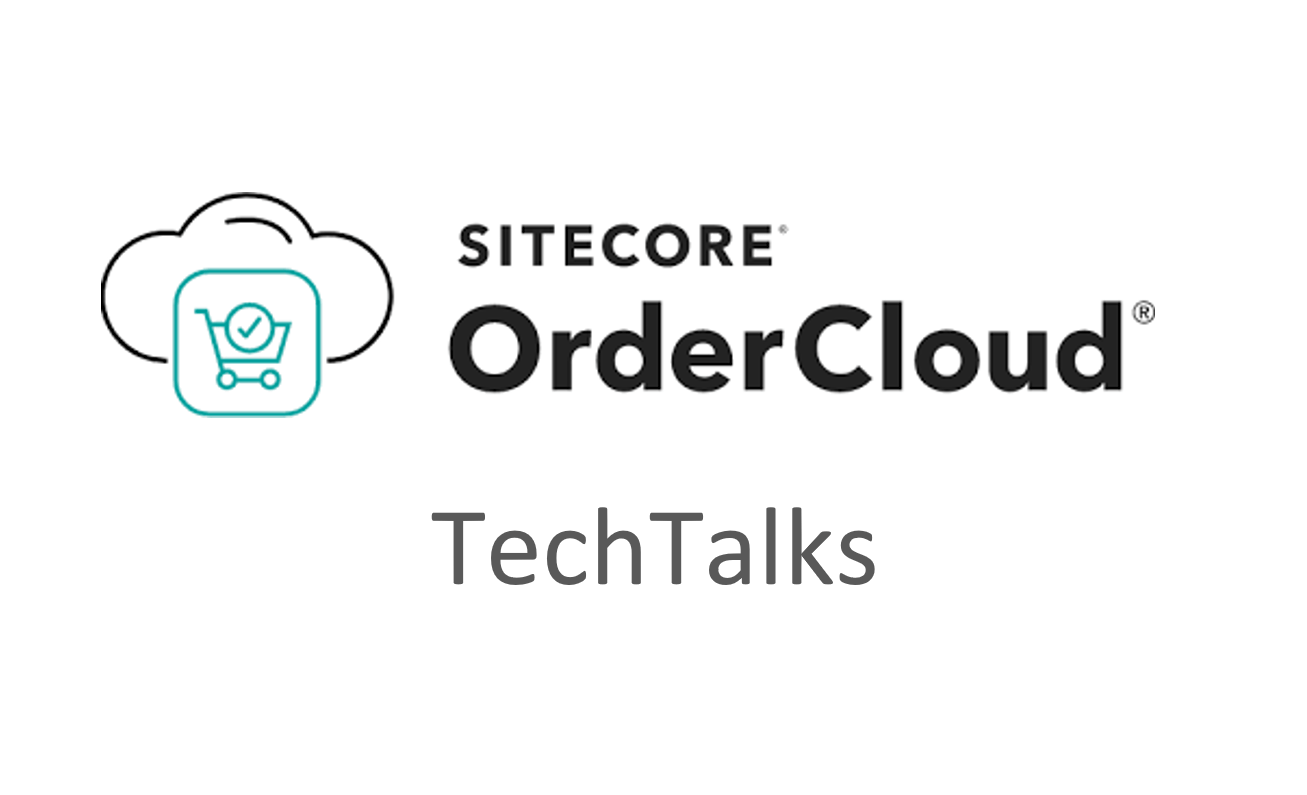
Guest Checkout in OrderCloud
Sitecore OrderCloud offers out-of-the-box support for guest checkout. You can enable this feature at the API Client level, which will require creating and assigning a default buyer user that’s shared across all guest customers. In practice, this means that every guest customer will use the same default buyer user credentials.
The key challenge is distinguishing between registered and anonymous customers when they interact with your custom middleware. Fortunately, OrderCloud provides a clear way to make this distinction using JWT token property.
Recognizing Anonymous vs. Registered Users
When a customer is anonymous, their JWT token includes an
property. This property is only present in tokens for guest or anonymous users. For example, when decoding an anonymous buyer user’s token, you’ll see the orderid
property:orderid
In contrast, tokens for registered users will not include this
property. A registered buyer's token will look like this:orderid
OrderCloud uses this
property to establish a link between the default guest user context and the unsubmitted order (the shopping cart). By checking for the presence or absence of the orderid
ordered
property, you can easily determine whether a user is a guest or a registered customer.
Creating a Filter for Custom Middleware
Based on the presence of the
property in the token, you can create a custom attribute (filter) for your middleware endpoint. This filter can inspect the token and decide whether to allow access. If the orderid
property exists, you know that the request is coming from an anonymous customer and can restrict access accordingly. If the property is absent, the user is registered, and you can permit them to access the endpoint.orderid
Here's a quick breakdown of the process:
- Decode the JWT token.
- Check for the
property.orderid
- If present, block the anonymous customer from accessing the middleware.
- If absent, allow registered users to proceed.
This simple approach ensures that only logged-in users can access specific features, improving the security and functionality of your ecommerce platform.
Here the filter/attribute example code:
public class RegisteredUserAttribute : ActionFilterAttribute
{
public override async Task OnActionExecutionAsync(ActionExecutingContext context, ActionExecutionDelegate next)
{
var headers = context.HttpContext.Request.Headers;
var decodedToken = GetUserToken(headers);
var isRegistered = string.IsNullOrEmpty(decodedToken.AnonOrderID);
Require.That(isRegistered, new ErrorCode("User is not registered", "User is not registered user", HttpStatusCode.Forbidden));
await next();
}
private DecodedToken GetUserToken(IHeaderDictionary headerDictionary)
{
var authorizationHeader = headerDictionary.Authorization.ToString();
var strArray = authorizationHeader?.Split(' ', 2);
Require.That(strArray != null && strArray[0].Equals("Bearer", StringComparison.OrdinalIgnoreCase), new UnauthorizedAccessException());
var token = strArray?[1].Trim();
return new DecodedToken(token);
}
}
I hope this guide helps you implement custom middleware restrictions in your Sitecore OrderCloud project. Happy coding!